Student Award
The Student Award section aims at recognizing the contributions of the students and showcasing their achievements. This section is situated below the Hero Section of TNP Home Page.
Creating Achievement and Award Slides
In order to implement Student Award Section, we need to create react functional components for both achievement and award slides.
The following steps are executed:
Opening the StudentAward.jsx file.
All the dependencies and relevant modules are imported.
StudentAward.jsximport { Swiper, SwiperSlide } from "swiper/react";
import {
Navigation,
Pagination,
Scrollbar,
A11y,
FreeMode,
Autoplay,
} from "swiper";
import "swiper/css";
import "swiper/css/navigation";
import "swiper/css/pagination";
import "swiper/css/scrollbar";
import "swiper/css/free-mode";A
StudentAward
component is structured as below:StudentAward.jsxconst StudentAward = () => {
// Component logic goes here
return (
<div className=" gap-4 flex flex-col md:flex-row">
{/* Render Swiper components */}
</div>
);
};Two data arrays,
achievementSlideObj
andawardSlideObj
are defined in the above component to store and edit data for achievement and award slides.StudentAward.jsxconst achievementSlideObj = [
studentImg: //image comes here,
achievementType: //define type of achievement,
studentName: //student name comes here,
caption: //Description of the achievement,
];
const awardSlideObj = [
awardImg: //image comes here,
awardName: //Name of the award,
caption: //Description comes here,
];In the next step, JSX elements for
achievementSlides
are defined using theachievementSlideObj
data and the structure is customized as needed.StudentAward.jsxconst achivementSlides = achievementSlideObj.map((data) => {
return (
<SwiperSlide key={data.studentName}>
{/* Render achievement slide content */}
</SwiperSlide>
);
});In the same way,
awardSlides
usingawardSlideObj
data are defined.StudentAward.jsxconst awardSlides = awardSlideObj.map((data) => {
return (
<SwiperSlide key={data.awardName}>
{/* Render award slide content */}
</SwiperSlide>
);
});
To understand more about JSX, refer the JSX documentation properly.
Swiper Components by passing the JSX for achievements and award slides are defined in this step. This is done in the following way:
StudentAward.jsxconst StudentAward = () => {
// Component logic goes here
return (
<div className="gap-4 flex flex-col md:flex-row">
{/*Left section*/}
/* Swiper for Awards */
<Swiper className="h-auto w-full lg:w-[48%] border rounded-lg xl:rounded-xl"
modules={[Navigation, A11y, Autoplay]}
spaceBetween={30}
slidesPerView={1}
navigation={...}>
{awardSlides}
</Swiper>
{/*Right Section*/}
{/* Swiper for Achievements */}
<Swiper className="h-auto w-full lg:w-[48%] border rounded-lg xl:rounded-xl"
modules={[Autoplay]}
spaceBetween={30}
slidesPerView={1}
navigation={...}>
{achivementSlides}
</Swiper>
</div>
);
};Component's appearance and functionality are customized according to the requirements.
Save the file.
Run
yarn dev
to see the changes in your local system.
The above steps results into the following output:
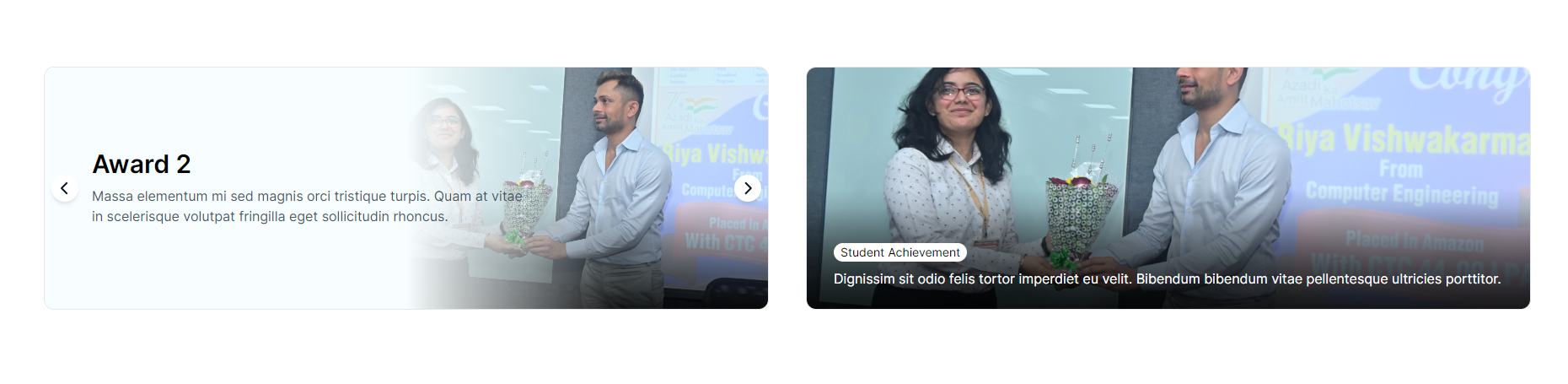
In this page, we saw how the Student Awards section was put together. Let's go on towards the next stage, the Testimonials Page, to see how the component was implemented.